Deploy a sample smart contract to Semita Application Chain
Write the Smart Contract Code: The first step is to write the smart contract code in a high-level programming language like Solidity, Vyper, or others. This code will be compiled into bytecode that can be executed by the EVM.
- Compile the Smart Contract Code: Once you have written the smart contract code, you need to compile it using a compiler such as the Solidity compiler. The compiler will produce the bytecode that can be deployed to the EVM.
- Connect to the Application Chain Network: To deploy the smart contract, you need to connect to an Application Chain network.
- Create a Wallet: You need to create a wallet that will be used to deploy the smart contract to the Application Chain network. You can use a wallet such as Metamask, MyEtherWallet, or others.
- Deploy the Smart Contract: Once you have connected to the Semita Application Chain network and created a wallet, you can deploy the smart contract using a tool such as Remix, Truffle, or others. You will need to specify the bytecode of the smart contract and the gas limit for the deployment. The deployment will create a transaction on the Semita Application Chain network that will be broadcast to the network for validation and inclusion in a block.
- Verify the Smart Contract: After deploying the smart contract, you may want to verify its source code on a blockchain explorer. This will allow others to see the source code of the smart contract and verify that it matches the deployed bytecode.
Deploy Sample Contract to Semita Application Chain
Below is an example of a smart contract that allows users to set and retrieve the message.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SampleContract {
string public message;
constructor(string memory _message) {
message = _message;
}
function setMessage(string memory _newMessage) public {
message = _newMessage;
}
}
This contract creates a simple storage for a message that can be set and retrieved. The message variable is declared as public, which means that it can be read by anyone. The setMessage function allows anyone to update the message variable.
The constructor function sets the initial value of the message variable when the contract is deployed to the Application Chain network. The memory keyword indicates that the argument is stored in memory, rather than in storage on the blockchain.
To deploy this contract, you would need to compile the code into bytecode and then deploy it to the Application Chain network using a tool such as Remix or Truffle. Once deployed, you could interact with the contract using a web3 provider such as MetaMask or a blockchain explorer.
Go to the Remix IDE, and create the Message.sol file and copy the example to the file.
- Compile the Solidity code.
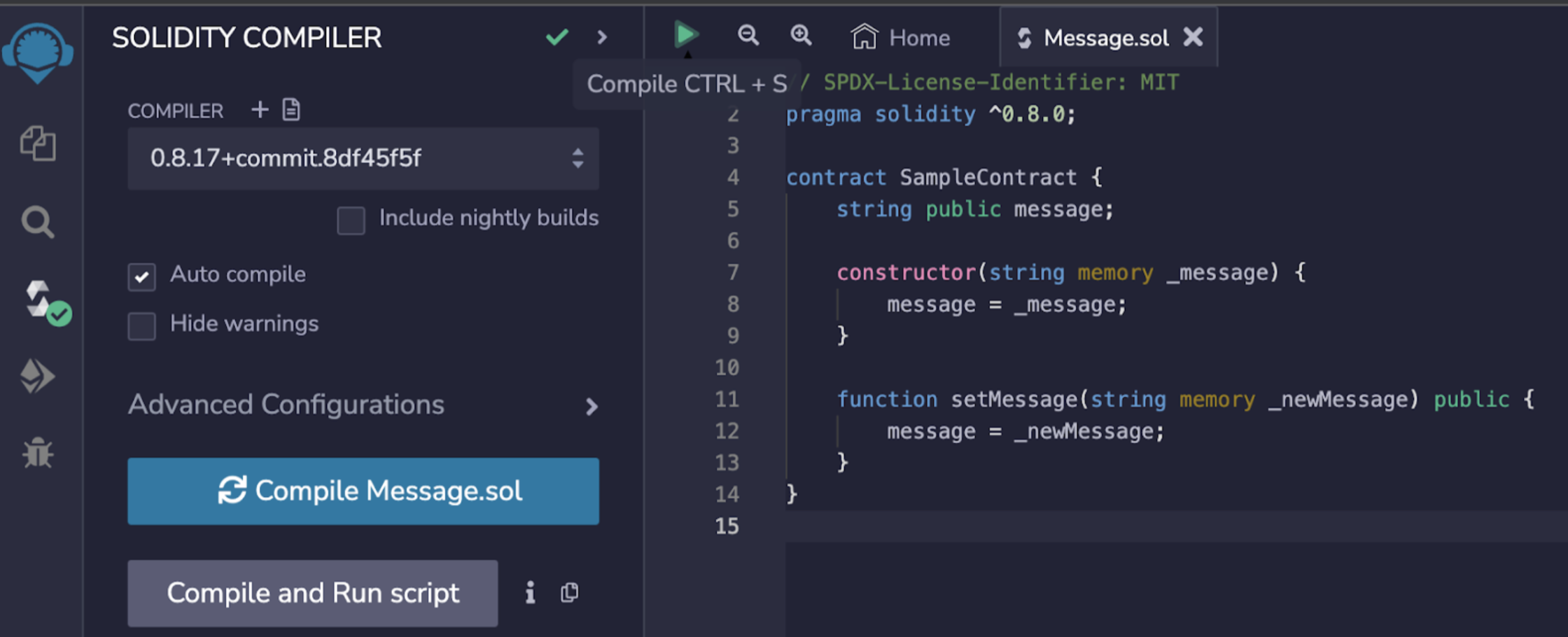
- Configure your Metamask with the network info provided. Go to Metamask Settings and Add a Network.
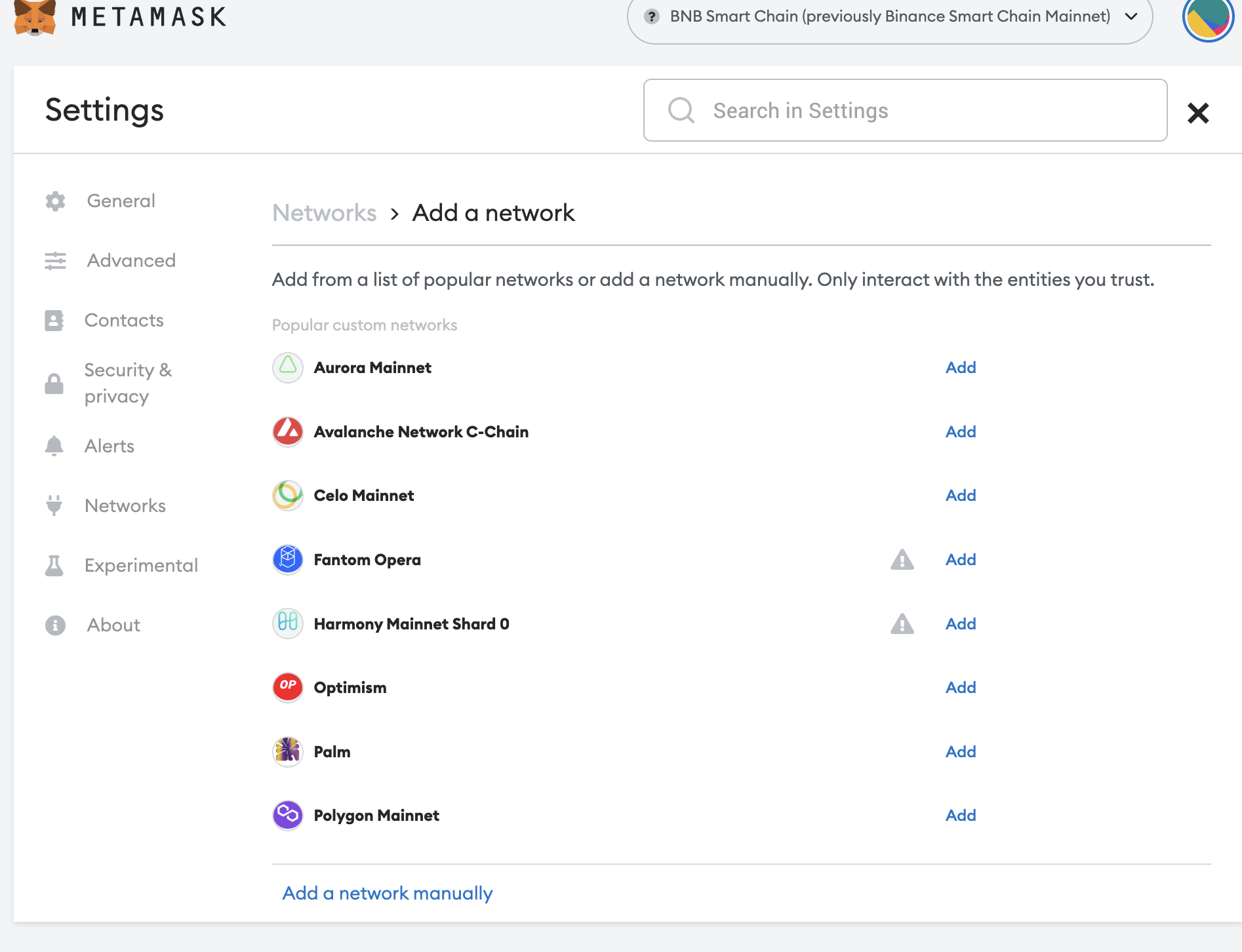
-
Select “Add a network manually”, and enter the corresponding information.
-
And switch your network to the Vodafone Blockchain.
-
Take tokens from the faucet, and you will see them in your wallet.
-
Configure your wallet in Remix.
Please note the environment need to select the “Injected Provider-MetaMask”, enter your public address, and click deploy. At the same time, your wallet will notify you to confirm the transaction to deploy the contract and click confirm to continue.
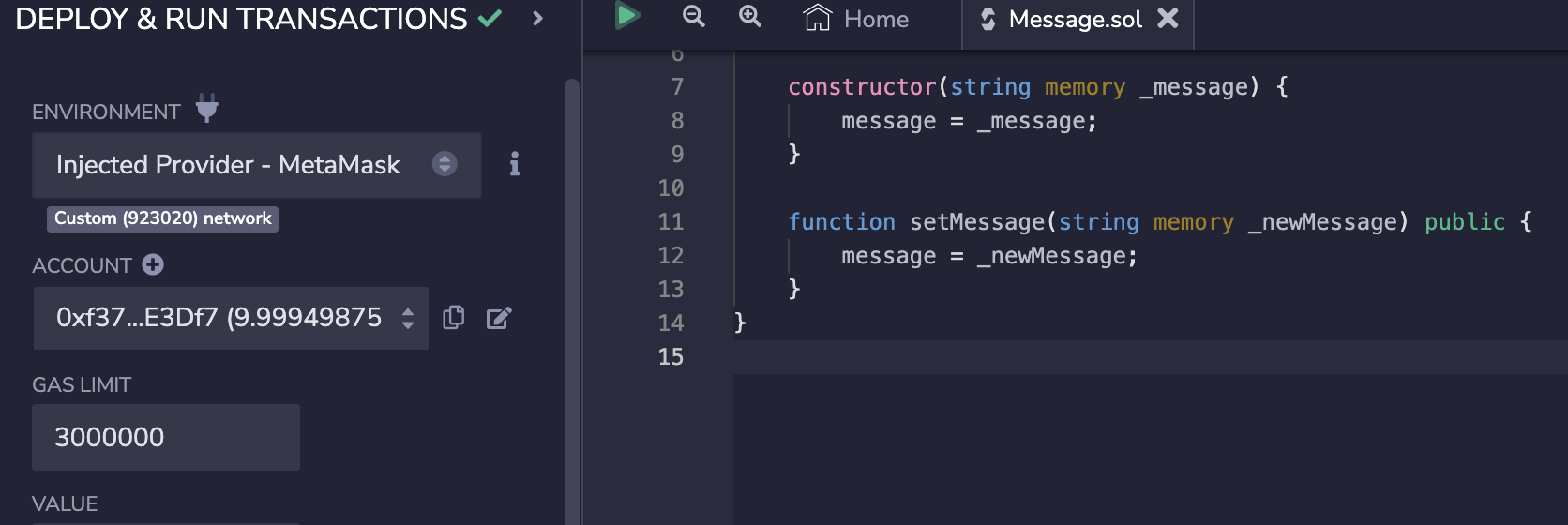
- Test the deployed contract.
In the deployed contracts section, enter the message you want to send. For example, “Hello Application Chain” and click send Message. You need to confirm the transaction on your wallet again to pay for the gas fee.
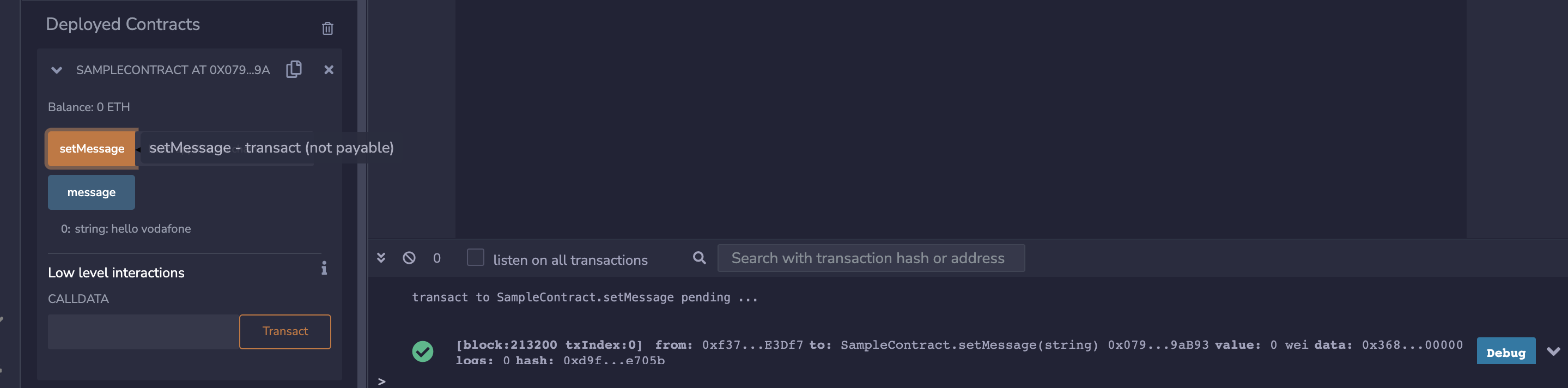
- Retrieve the message.
In the “Deployed Contracts” section, click the message to retrieve.
Updated about 2 years ago